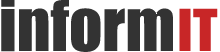

Author: Stephen Prata
Publisher: Addison-Wesley Professional
ISBN: 978-0-13-431061-9
Copyright © 2012 by Pearson Education, Inc.
Contents at a Glance
Introduction
1. Getting Started with C++
2. Setting Out to C++
3. Dealing with Data
4. Compound Types
5. Loops and Relational Expressions
6. Branching Statements and Logical Operators
7. Functions: C++’s Programming Modules
8. Adventures in Functions
9. Memory Models and Namespaces
10. Objects and Classes
11. Working with Classes
12. Classes and Dynamic Memory Allocation
13. Class Inheritance
14. Reusing Code in C++
15. Friends, Exceptions, and More
16. The string
Class and the Standard Template Library
17. Input, Output, and Files
18. Visiting with the New C++ Standard
Appendixes
A. Number Bases
B. C++ Reserved Words
C. The ASCII Character Set
D. Operator Precedence
E. Other Operators
F. The string
Template Class
G. The Standard Template Library Methods and Functions
H. Selected Readings and Internet Resources
I. Converting to ISO Standard C++
Introduction
Learning C++ is an adventure of discovery, particularly because the language accommodates several programming paradigms, including object-oriented programming, generic programming, and the traditional procedural programming. The fifth edition of this book described the language as set forth in the ISO C++ standards, informally known as C++99 and C++03, or, sometimes as C++99/03. (The 2003 version was largely a technical correction to the 1999 standard and didn’t add any new features.) Since then, C++ continues to evolve. As this book is written, the international C++ Standards Committee has just approved a new version of the standard. This standard had the informal name of C++0x while in development, and now it will be known as C++11. Most contemporary compilers support C++99/03 quite well, and most of the examples in this book comply with that standard. But many features of the new standard already have appeared in some implementations, and this edition of C++ Primer Plus explores these new features.
C++ Primer Plus discusses the basic C language and presents C++ features, making this book self-contained. It presents C++ fundamentals and illustrates them with short, to-the-point programs that are easy to copy and experiment with. You learn about input/output (I/O), how to make programs perform repetitive tasks and make choices, the many ways to handle data, and how to use functions. You learn about the many features C++ has added to C, including the following:
- Classes and objects
- Inheritance
- Polymorphism, virtual functions, and runtime type identification (RTTI)
- Function overloading
- Reference variables
- Generic, or type-independent, programming, as provided by templates and the Standard Template Library (STL)
- The exception mechanism for handling error conditions
- Namespaces for managing names of functions, classes, and variables
The Primer Approach
C++ Primer Plus brings several virtues to the task of presenting all this material. It builds on the primer tradition begun by C Primer Plus nearly two decades ago and embraces its successful philosophy:
- A primer should be an easy-to-use, friendly guide.
- A primer doesn’t assume that you are already familiar with all relevant programming concepts.
- A primer emphasizes hands-on learning with brief, easily typed examples that develop your understanding, a concept or two at a time.
- A primer clarifies concepts with illustrations.
- A primer provides questions and exercises to let you test your understanding, making the book suitable for self-learning or for the classroom.
Following these principles, the book helps you understand this rich language and how to use it. For example
- It provides conceptual guidance about when to use particular features, such as using public inheritance to model what are known as is-a relationships.
- It illustrates common C++ programming idioms and techniques.
- It provides a variety of sidebars, including tips, cautions and notes.
The author and editors of this book do our best to keep the presentation to-the-point, simple, and fun. Our goal is that by the end of the book, you’ll be able to write solid, effective programs and enjoy yourself doing so.
Sample Code
This book provides an abundance of sample code, most of it in the form of complete programs. Like the previous editions, this book practices generic C++ so that it is not tied to any particular kind of computer, operating system, or compiler. Thus, the examples were tested on a Windows 7 system, a Macintosh OS X system, and a Linux system. Those programs using C++11 features require compilers supporting those features, but the remaining programs should work with any C++99/03-compliant system.
The sample code for the complete programs described in this book is available on this book’s website. See the registration link given on the back cover for more information.
Organization
This book is divided into 18 chapters and 10 appendixes, summarized here:
- Chapter 1: Getting Started with C++— Chapter 1 relates how Bjarne Stroustrup created the C++ programming language by adding object-oriented programming support to the C language. You’ll learn the distinctions between procedural languages, such as C, and object-oriented languages, such as C++. You’ll read about the joint ANSI/ISO work to develop a C++ standard. This chapter discusses the mechanics of creating a C++ program, outlining the approach for several current C++ compilers. Finally, it describes the conventions used in this book.
- Chapter 2: Setting Out to C++— Chapter 2 guides you through the process of creating simple C++ programs. You’ll learn about the role of the
main()
function and about some of the kinds of statements that C++ programs use. You’ll use the predefined cout
and cin
objects for program output and input, and you’ll learn about creating and using variables. Finally, you’ll be introduced to functions, C++’s programming modules.
- Chapter 3: Dealing with Data— C++ provides built-in types for storing two kinds of data: integers (numbers with no fractional parts) and floating-point numbers (numbers with fractional parts). To meet the diverse requirements of programmers, C++ offers several types in each category. Chapter 3 discusses those types, including creating variables and writing constants of various types. You’ll also learn how C++ handles implicit and explicit conversions from one type to another.
- Chapter 4: Compound Types— C++ lets you construct more elaborate types from the basic built-in types. The most advanced form is the class, discussed in Chapters 9 through 13. Chapter 4 discusses other forms, including arrays, which hold several values of a single type; structures, which hold several values of unlike types; and pointers, which identify locations in memory. You’ll also learn how to create and store text strings and to handle text I/O by using C-style character arrays and the C++
string
class. Finally, you’ll learn some of the ways C++ handles memory allocation, including using the new
and delete
operators for managing memory explicitly.
- Chapter 5: Loops and Relational Expressions— Programs often must perform repetitive actions, and C++ provides three looping structures for that purpose: the
for
loop, the while
loop, and the do while
loop. Such loops must know when they should terminate, and the C++ relational operators enable you to create tests to guide such loops. In Chapter 5 you learn how to create loops that read and process input character-by-character. Finally, you’ll learn how to create two-dimensional arrays and how to use nested loops to process them.
- Chapter 6: Branching Statements and Logical Operators— Programs can behave intelligently if they can tailor their behavior to circumstances. In Chapter 6 you’ll learn how to control program flow by using the
if
, if else
, and switch
statements and the conditional operator. You’ll learn how to use logical operators to help express decision-making tests. Also, you’ll meet the cctype
library of functions for evaluating character relations, such as testing whether a character is a digit or a nonprinting character. Finally, you’ll get an introductory view of file I/O.
- Chapter 7: Functions: C++’s Programming Modules— Functions are the basic building blocks of C++ programming. Chapter 7 concentrates on features that C++ functions share with C functions. In particular, you’ll review the general format of a function definition and examine how function prototypes increase the reliability of programs. Also, you’ll investigate how to write functions to process arrays, character strings, and structures. Next, you’ll learn about recursion, which is when a function calls itself, and see how it can be used to implement a divide-and-conquer strategy. Finally, you’ll meet pointers to functions, which enable you to use a function argument to tell one function to use a second function.
- Chapter 8: Adventures in Functions— Chapter 8 explores the new features C++ adds to functions. You’ll learn about inline functions, which can speed program execution at the cost of additional program size. You’ll work with reference variables, which provide an alternative way to pass information to functions. Default arguments let a function automatically supply values for function arguments that you omit from a function call. Function overloading lets you create functions having the same name but taking different argument lists. All these features have frequent use in class design. Also you’ll learn about function templates, which allow you to specify the design of a family of related functions.
- Chapter 9: Memory Models and Namespaces— Chapter 9 discusses putting together multifile programs. It examines the choices in allocating memory, looking at different methods of managing memory and at scope, linkage, and namespaces, which determine what parts of a program know about a variable.
- Chapter 10: Objects and Classes— A class is a user-defined type, and an object (such as a variable) is an instance of a class. Chapter 10 introduces you to object-oriented programming and to class design. A class declaration describes the information stored in a class object and also the operations (class methods) allowed for class objects. Some parts of an object are visible to the outside world (the public portion), and some are hidden (the private portion). Special class methods (constructors and destructors) come into play when objects are created and destroyed. You will learn about all this and other class details in this chapter, and you’ll see how classes can be used to implement ADTs, such as a stack.
- Chapter 11: Working with Classes— In Chapter 11 you’ll further your understanding of classes. First, you’ll learn about operator overloading, which lets you define how operators such as
+
will work with class objects. You’ll learn about friend functions, which can access class data that’s inaccessible to the world at large. You’ll see how certain constructors and overloaded operator member functions can be used to manage conversion to and from class types.
- Chapter 12: Classes and Dynamic Memory Allocation— Often it’s useful to have a class member point to dynamically allocated memory. If you use
new
in a class constructor to allocate dynamic memory, you incur the responsibilities of providing an appropriate destructor, of defining an explicit copy constructor, and of defining an explicit assignment operator. Chapter 12 shows you how and discusses the behavior of the member functions generated implicitly if you fail to provide explicit definitions. You’ll also expand your experience with classes by using pointers to objects and studying a queue simulation problem.
- Chapter 13: Class Inheritance— One of the most powerful features of object-oriented programming is inheritance, by which a derived class inherits the features of a base class, enabling you to reuse the base class code. Chapter 13 discusses public inheritance, which models is-a relationships, meaning that a derived object is a special case of a base object. For example, a physicist is a special case of a scientist. Some inheritance relationships are polymorphic, meaning you can write code using a mixture of related classes for which the same method name may invoke behavior that depends on the object type. Implementing this kind of behavior necessitates using a new kind of member function called a virtual function. Sometimes using abstract base classes is the best approach to inheritance relationships. This chapter discusses these matters, pointing out when public inheritance is appropriate and when it is not.
- Chapter 14: Reusing Code in C++— Public inheritance is just one way to reuse code. Chapter 14 looks at several other ways. Containment is when one class contains members that are objects of another class. It can be used to model has-a relationships, in which one class has components of another class. For example, an automobile has a motor. You also can use private and protected inheritance to model such relationships. This chapter shows you how and points out the differences among the different approaches. Also, you’ll learn about class templates, which let you define a class in terms of some unspecified generic type, and then use the template to create specific classes in terms of specific types. For example, a stack template enables you to create a stack of integers or a stack of strings. Finally, you’ll learn about multiple public inheritance, whereby a class can derive from more than one class.
- Chapter 15: Friends, Exceptions, and More— Chapter 15 extends the discussion of friends to include friend classes and friend member functions. Then it presents several new developments in C++, beginning with exceptions, which provide a mechanism for dealing with unusual program occurrences, such an inappropriate function argument values and running out of memory. Then you’ll learn about RTTI, a mechanism for identifying object types. Finally, you’ll learn about the safer alternatives to unrestricted typecasting.
- Chapter 16: The
string
Class and the Standard Template Library— Chapter 16 discusses some useful class libraries recently added to the language. The string
class is a convenient and powerful alternative to traditional C-style strings. The auto_ptr
class helps manage dynamically allocated memory. The STL provides several generic containers, including template representations of arrays, queues, lists, sets, and maps. It also provides an efficient library of generic algorithms that can be used with STL containers and also with ordinary arrays. The valarray
template class provides support for numeric arrays.
- Chapter 17: Input, Output, and Files— Chapter 17 reviews C++ I/O and discusses how to format output. You’ll learn how to use class methods to determine the state of an input or output stream and to see, for example, whether there has been a type mismatch on input or whether the end-of-file has been detected. C++ uses inheritance to derive classes for managing file input and output. You’ll learn how to open files for input and output, how to append data to a file, how to use binary files, and how to get random access to a file. Finally, you’ll learn how to apply standard I/O methods to read from and write to strings.
- Chapter 18: Visiting with the New C++ Standard— Chapter 18 begins by reviewing several C++11 features introduced in earlier chapters, including new types, uniform initialization syntax, automatic type deduction, new smart pointers, and scoped enumerations. The chapter then discusses the new rvalue reference type and how it’s used to implement a new feature called move semantics. Next, the chapter covers new class features, lambda expressions, and variadic templates. Finally, the chapter outlines many new features not covered in earlier chapters of the book.
- Appendix A: Number Bases— Appendix A discusses octal, hexadecimal, and binary numbers.
- Appendix B: C++ Reserved Words— Appendix B lists C++ keywords.
- Appendix C: The ASCII Character Set— Appendix C lists the ASCII character set, along with decimal, octal, hexadecimal, and binary representations.
- Appendix D: Operator Precedence— Appendix D lists the C++ operators in order of decreasing precedence.
- Appendix E: Other Operators— Appendix E summarizes the C++ operators, such as the bitwise operators, not covered in the main body of the text.
- Appendix F: The
string
Template Class— Appendix F summarizes string class methods and functions
.
- Appendix G: The Standard Template Library Methods and Functions— Appendix G summarizes the STL container methods and the general STL algorithm functions.
- Appendix H: Selected Readings and Internet Resources— Appendix H lists some books that can further your understanding of C++.
- Appendix I: Converting to ISO Standard C++— Appendix I provides guidelines for moving from C and older C++ implementations to ANSI/ISO C++.
Special Features in the Learning Lab Web Edition
This online Learning Lab edition of the book includes several unique special features:
- The complete text of the book online so you can read at your own pace, whenever you want
- Live code-editing sandboxes that let you try each of the examples yourself and see the results with the click of a button
- Easy-to-follow review videos that show you how perform key tasks in each chapter
- Fully interactive quizzes to test your understanding of the material after each lesson
- Online exercises that let you expand on what you've learned in a chapter and stretch your programming skills
Conventions Used
This book uses several typographic conventions to distinguish among various kinds of text:
- Code lines, commands, statements, variables, filenames, and program output appear in a
computer typeface:
#include <iostream>
int main()
{
using namespace std;
cout << "What's up, Doc!\n";
return 0;
}
- Program input that you should type appears in
bold computer typeface:
Please enter your name:
Plato
- Placeholders in syntax descriptions appear in an
italic computer typeface
. You should replace a placeholder with the actual filename, parameter, or whatever element it represents.
- Italic type is used for new terms.
Tip
Tips present short, helpful guides to particular programming situations.
Caution
A caution alerts you to potential pitfalls.
Note
The notes provide a catch-all category for comments that don’t fall into one of the other categories.
Systems Used to Develop the Programming Examples
For the record, the C++11 examples in this book were developed using Microsoft Visual C++ 2010 and Cygwin with Gnu g++ 4.5.0, both running under 64-bit Windows 7. The remaining examples were tested with these systems, as well as on an iMac using g++ 4.2.1 under OS X 10.6.8 and on an Ubuntu Linux system using g++ 4.4.1. Most of the pre-C++11 examples were originally developed using Microsoft Visual C++ 2003 and Metrowerks CodeWarrior Development Studio 9 running under Windows XP Professional and checked using the Borland C++ 5.5 command-line compiler and GNU gpp
3.3.3 on the same system, using Comeau 4.3.3 and GNU g++ 3.3.1 under SuSE 9.0 Linux, and using Metrowerks Development Studio 9 on a Macintosh G4 under OS 10.3.
C++ offers a lot to the programmer; learn and enjoy!
About the Author
Stephen Prata taught astronomy, physics, and computer science at the College of Marin in Kentfield, California. He received his B.S. from the California Institute of Technology and his Ph.D. from the University of California, Berkeley. He has authored or coauthored more than a dozen books on programming topics including New C Primer Plus, which received the Computer Press Association’s 1990 Best How-to Computer Book Award, and C++ Primer Plus, nominated for the Computer Press Association’s Best How-to Computer Book Award in 1991.